This post show how to create GUI using
Glade, and link the generated glade file in c code, then compile with gcc with GTK+ 3.0, to generate a executable program.
- Start glade, create something. Specify the window in TOPLEVEL with name "myname". Save as "testGlade.glade". We will refer them in our c code.
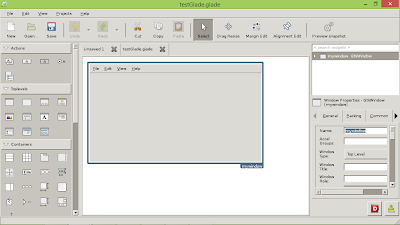 |
Create GUI with Glade |
- Create our c source code, "test.c".
#include <gtk/gtk.h>
int main(int argc, char *argv[])
{
GtkBuilder *gtkBuilder;
GtkWidget *window;
gtk_init(&argc, &argv);
gtkBuilder = gtk_builder_new();
gtk_builder_add_from_file(gtkBuilder, "testGlade.glade", NULL);
window = GTK_WIDGET(gtk_builder_get_object(gtkBuilder, "mywindow"));
g_object_unref(G_OBJECT(gtkBuilder));
gtk_widget_show(window);
gtk_main();
return 0;
}
where "mywindow" is the window defined in Glade. And "testGlade.glade" is our saved glade file.
- Compile it with command:
$
gcc -Wall -g -o testGlade test.c `pkg-config --cflags --libs gtk+-3.0`
Where testGlade is the target executable file. Run it:
$
./testGlade
Because we have do nothing on the code. The program just show a menu bar without action.
Remark:
It's commented to use following command:
gcc -Wall -g -o testGlade test.c $(pkg-config --cflags gtk+-3.0) $(pkg-config --libs gtk+-3.0)
~ thx teknikkim